You have to hide under a rock to not hear anything about ChatGPT, Generative AI, LLM’s, etc. They are all over the place. It’s hard to not be confronted with these technologies. There are multiple ways to look at these technologies. You either see it as a thread and try to avoid using it. The downside of technological innovations is that people with bad intentions can benefit from it too. Next to that technological innovations always seems to have impact on various jobs. Are these jobs disappearing? Some of them probably will. Other jobs might become more interesting because these innovations might be able to automate some routine tasks. The benefit of that I that there is more time for other, potentially more interesting, tasks. In these cases it might be wise to embrace technological innovation and see how you can benefit from this.
ChatGPT
When it comes to ChatGPT, tons of articles, blogs and books are written. With or without the help/ support of ChatGPT itself. I am no expert in ChatGPT, but I use it on regular basis to see how it can make my life more easy. One thing I learned along the way is; the better you specify your question, the better ChatGPT’s answer. One possible way to specify these prompts is by using the 5W1H Method. Using this method, you construct a prompt via answers to the following 6 questions; who?, what?, where?, why?, when? and how?
5W1H Method
According to ChatGPT, the 5W1H Method should give you the following insight:
- Who? — Understanding “who” can help in tailoring the language and complexity of the response.
- What? — Specifying “what” ensures that the AI provides the type and format of information you desire.
- Where? — Defining “where” can help in receiving region or context-specific answers.
- Why? — Knowing “why” can help in determining the depth and angle of the AI’s response.
- When? — Framing “when” can help narrow down the context of the information provided.
- How? — Clarifying “how” can guide the AI in structuring its answer in the most useful way.
Asking ChatGPT Anything
The goal of this blog is to create a Streamlit Application and using the OpenAI API to send prompts, constructed using the 5W1H Method, to ChatGPT.
Application Setup
Before creating the actual Streamlit Application, we need to perform a few setup/ configuration steps.
- Generate OpenAI API Key
- Add Environment Variable
- OPENAI_API_KEY
- Add Environment Variable
- Choose ChatGPT Engine Model
- Install required packages
- Streamlit
- OpenAI
- Additional packages
1 – Generate OpenAI API Key
To be able to chat with ChatGPT via the OpenAI API, you need to have a OpenAI API Key for authentication. You can generate one by setting up an account on; https://platform.openai.com.
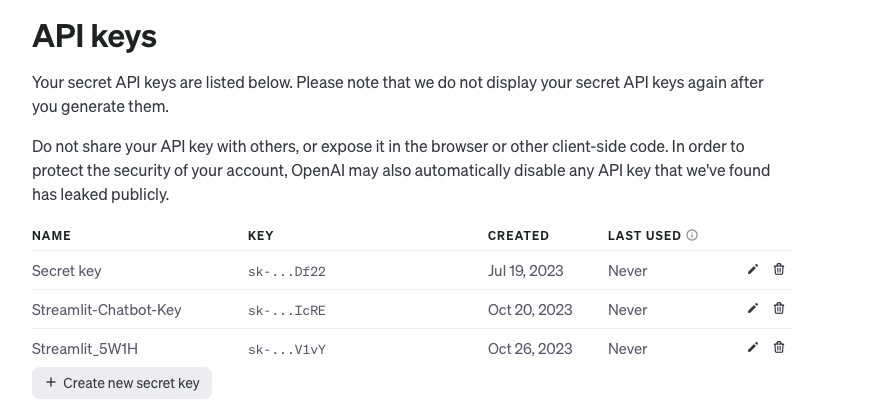
I created an environment file where I stored the OpenAI API Key. This file can be referenced in the Streamlit Application. This way you do not have to store the OpenAI API Key in the Streamlit Python file.
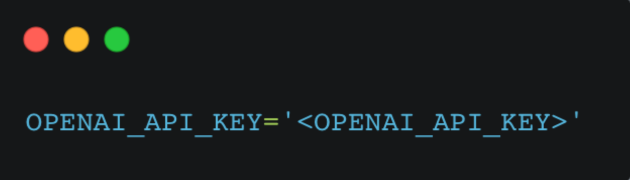
2 – Choose ChatGPT Engine Model
Choose the Model Engine you want to use in the Streamlit Application. I chose the ‘gpt-3.5-turbo’ Engine Model. According to ChatGPT; “The gpt-3.5-turbo
is a versatile and cost-efficient engine from OpenAI, designed for a broad range of applications including multi-turn conversations. It offers a balance of performance and cost, making it ideal for tasks like content generation and building chatbots.”
3 – Install required packages
I use a Conda environment where I create my Streamlit Application in. In this environment I install all the required packages; e.g. Streamlit, OpenAI and in my case Dotenv to load the OpenAI API Key Environment file.
Building the Streamlit Application
At this point everything is set for building the Streamlit Application.
- Import required packages
- Streamlit
- OpenAI
- Additional packages
- OpenAI configuration
- Set Page configuration
- Sidebar configuration (optional)
- Create OpenAI response function
- Submit the prompt_output to the OpenAI API
- Return the chat response
- Create Main Application
- Collecting user input according to the 5W1H Method
- Sending user input to ChatGPT function
- Display the ChatGPT response
4 – Import required packages
By default for this specific Streamlit Application there is a need to import the Streamlit and OpenAI packages. Next to that there are additional packages (Dotenv & Os) necessary to load en use the OpenAI API Key. Additionally the Re-package is required structure and format the ChatGPT-output
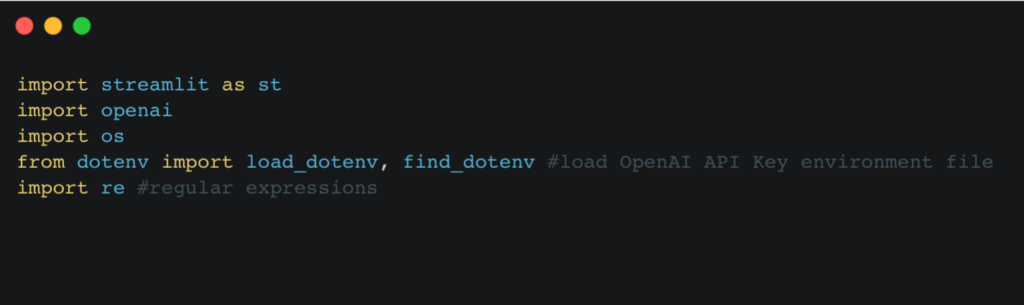
5 – OpenAI configuration
There are a few configuration steps necessary to be able to communicate with the OpenAI API:
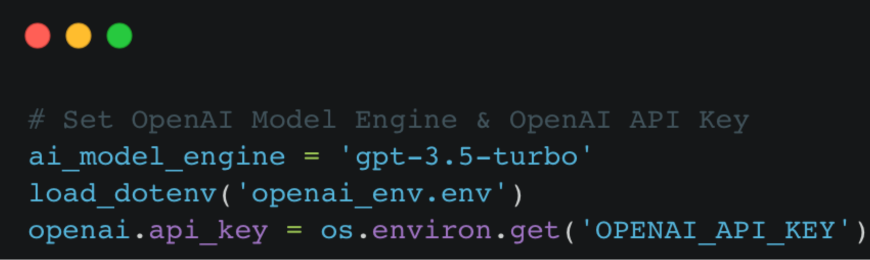
The following was not enough for this example; “openai.api_key = os.environ.get(‘OPENAI_API_KEY’)’. Therefore I needed to load the Environment-file with the Dotenv-package.
6 – Page configuration
The following two configurations are just to setup the page and optionally the sidebar. In this example there are two settings to set the page config (st.set_page_config) and the title (st.title).
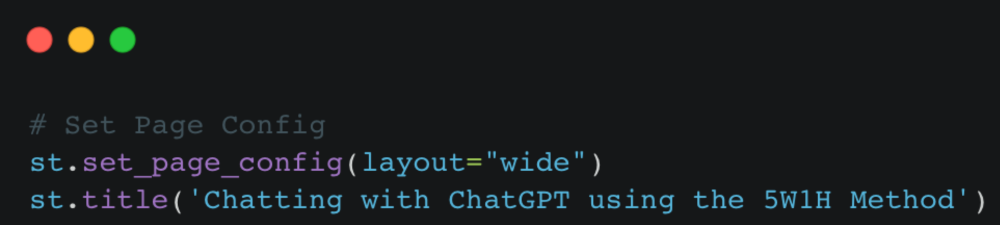
7 – Sidebar configuration (optional)
Including a sidebar in your Streamlit Application is just a matter of choice. In this example I just the sidebar to explain the 5W1H Method in short.
st.sidebar.markdown
- st = the Streamlit package
- sidebar = everything you want to put in the sidebar
- markdown = the option to use markdown
8 – OpenAI response function
Use the OpenAI library to generate a response based on the prompt that will be constructed in the Main Application.
There are basically to steps in this function:
- Submit the prompt_output to the OpenAI API
- Return the chat response
The ChatCompletion function takes at least two input parameters:
- model – the Model Engine
- messages
According to the documentation; “Chat models take a list of messages as input and return a model-generated message as output. Although the chat format is designed to make multi-turn conversations easy, it’s just as useful for single-turn tasks without any conversation.”
The messages
parameter in the ChatCompletion
function of the OpenAI library is a list of message objects. Each message object typically has two properties: role
and content
. The role
can be one of three values: system
, user
, or assistant
.
Start with a system
message to set the tone or behavior (optional). Alternate between user
and assistant
messages to simulate a back-and-forth conversation. This example has just a single-turn task.
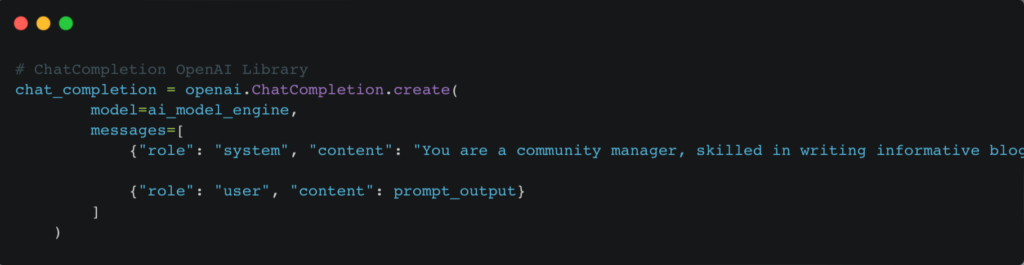
9 – Main Application
The Main Application consists of three parts:
- Collecting user input according to the 5W1H Method
- Sending user input to ChatGPT function
- Display the ChatGPT response
First, there are 6 text areas’s (st.text_area) so users can enter their answers based on the 5W1H Method. These answers are stored in 6 variables, which are concatenated as one variable.
Second, on submit (st.form & st.form_submit_button) this one variable is submitted to the OpenAI response function.
Third, in this example I defined the ‘How’ as; “I need a list of at least 10 topics. Can you provide such a list, highlighting why each topic is interesting, relevant, and current? Furthermore, please order the list based on the topics’ actuality and relevance.”. The function returns a list of topics and descriptions which I formatted with the help of ChatGPT.
ChatGPT also provide a breakdown of the regex pattern:
\d+\.
: Matches the numbering (e.g., “1.”, “2.”, etc.).(.*?):
: Captures the topic title until it finds a colon.\s
: Matches a space.(.*?)(?=\d+\.|$)
: Captures the description. The description continues until it either finds the next topic number or reaches the end of the string ($
). The(?=...)
part is a positive lookahead that ensures the description is captured until the next topic or the end, without consuming the next topic.
Using the re.S
flag makes the .
in the regex match newline characters as well, which ensures descriptions spanning multiple lines are captured correctly.
10 – Output
In this example I asked ChatGPT to help me find interesting topics to write about as a Snowflake Data Superhero. Please note that the version of ChatGPT I used (gpt-3.5-turbo) is trained with data up until September 2021. A lot has happened since. The returned topics are not necessarily interesting, relevant, and current like I asked.
The prompt I used according to the 5W1H Method and with a little bit of help of ChatGPT looks as follows…..
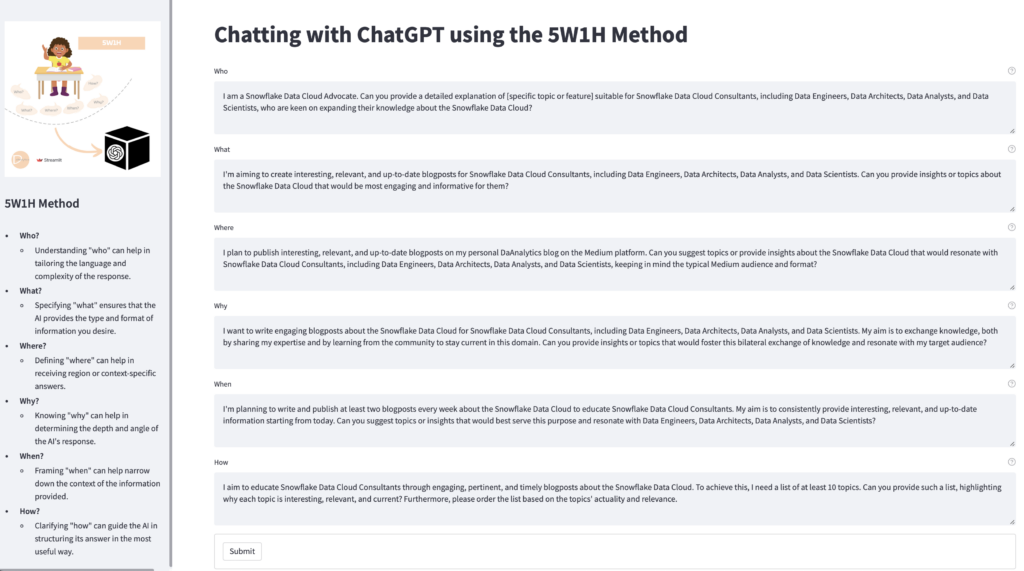
….. and gives the following result.
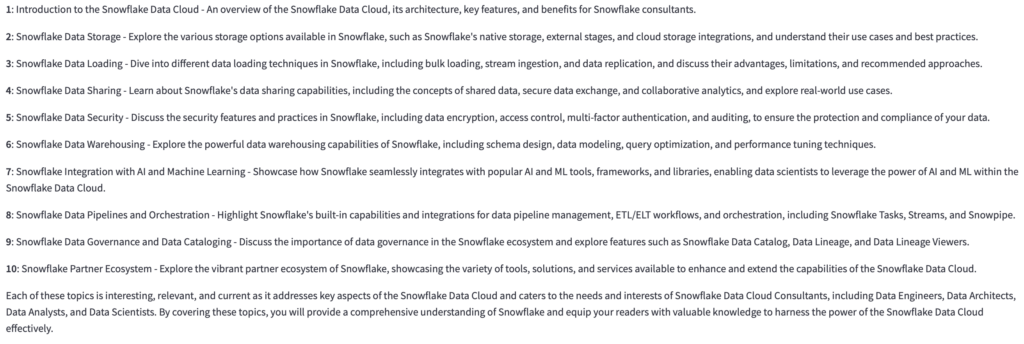
Summary
In this blog I showed how to use ChatGPT using the OpenAI library from Streamlit. The prompt to ask ChatGPT for output has been constructed using the 5W1H Method. This example has explicitly been created to work with a returned list of topics and descriptions. If you follow this example then you this code broadly shows how using the OpenAI library from Streamlit works. You als can get an idea of how to construct prompts following the 5W1H Method. It is by no means a on-size-fits-all solution.
Find all the code on my GitHub.
Till next time.
Snowflake Data Superhero. Online also known as; DaAnalytics.
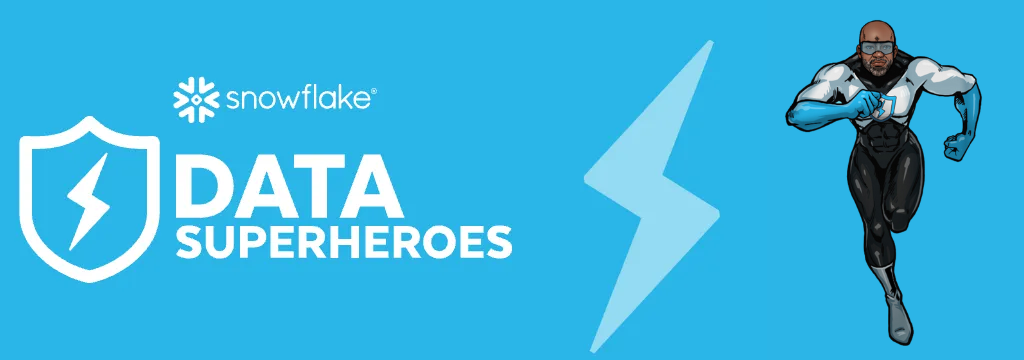